Ajax Load A Drupal View
Published:
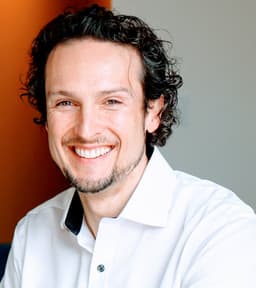
Heads up! This content is more than six months old. Take some time to verify everything still works as expected.
Had an interesting request today from multiple departments of the company. Here's short of them that's relevant.
- Marketing needed content that was optional heights, fixed width. This banner should link somewhere. It should come from a content type that can be easily maintained.
- SEO needed that same banner to have no seo value, which meant going through Iframe or Ajax.
Due to the complexity of the design, and need for ease of
maintenance, a view of a content type was perfect, but violated the
seo need. An iframe was mostly useless, due to the varied height of
the content. So, we prepared the follow code to ajax the views onto
the page after initial load. Note that this is only iteration one
for this technique. I could see it causing potential issues when
dealing with ajax views inserted into the page the standard way,
though I tried to accommodate that by changing the $view->dom_id
.
template.php
This works well if you expect to needs these views on every page.
/** * Ajax Load Views * See scripts/emfluence.js for the actual firing js **/ $views_ajax_settings = array( 'views' => array( 'ajax_path' => url('views/ajax'), 'ajaxViews' => array() ), ); $view = views_get_view('Home_Page_Banner'); $view->set_display('default'); $view->set_use_ajax(TRUE); $views_ajax_settings['views']['ajaxviews'][] = array( 'view_name' => $view->name, 'view_display_id' => $view->current_display, 'view_args' => check_plain(implode('/', $view->args)), 'view_path' => check_plain($\_GET['q']), 'view_base_path' => $view->get_path(), 'view_dom_id' => $view->name, 'pager_element' => \$view->pager['element'], ); $view = views_get_view('News'); $view->set_display('default'); $view->set_use_ajax(TRUE); $views_ajax_settings['views']['ajaxviews'][] = array( 'view_name' => $view->name, 'view_display_id' => $view->current_display, 'view_args' => check_plain(implode('/', $view->args)), 'view_path' => check_plain($\_GET['q']), 'view_base_path' => $view->get_path(), 'view_dom_id' => $view->name, 'pager_element' => \$view->pager['element'], ); drupal_add_js(\$views_ajax_settings, 'setting'); views_add_js('ajax_view');
Page.tpl.php
This method works on just the desired page, lessening the overhead of calling views you don't need through the whole site.
Note that you must still include views_add_js('ajax_view');
in
your template.php file!
/** * Ajax Load Views * See scripts/emfluence.js for the actual firing js **/ $views_ajax_settings = array( 'views' => array( 'ajax_path' => url('views/ajax'), 'ajaxViews' => array() ), ); $view = views_get_view('Home_Page_Banner'); $view->set_display('default'); $view->set_use_ajax(TRUE); $views_ajax_settings['views']['ajaxviews'][] = array( 'view_name' => $view->name, 'view_display_id' => $view->current_display, 'view_args' => check_plain(implode('/', $view->args)), 'view_path' => check_plain($\_GET['q']), 'view_base_path' => $view->get_path(), 'view_dom_id' => $view->name, 'pager_element' => \$view->pager['element'], ); $view = views_get_view('News'); $view->set_display('default'); $view->set_use_ajax(TRUE); $views_ajax_settings['views']['ajaxviews'][] = array( 'view_name' => $view->name, 'view_display_id' => $view->current_display, 'view_args' => check_plain(implode('/', $view->args)), 'view_path' => check_plain($\_GET['q']), 'view_base_path' => $view->get_path(), 'view_dom_id' => $view->name, 'pager_element' => \$view->pager['element'], ); $view = views_get_view('Home_Page_Spotlight'); $view->set_display('default'); $view->set_use_ajax(TRUE); $views_ajax_settings['views']['ajaxviews'][] = array( 'view_name' => $view->name, 'view_display_id' => $view->current_display, 'view_args' => check_plain(implode('/', $view->args)), 'view_path' => check_plain($\_GET['q']), 'view_base_path' => $view->get_path(), 'view_dom_id' => $view->name, 'pager_element' => \$view->pager['element'], );
<html> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="<?php print $language->language ?>" lang="<?php print $language->language ?>" dir="<?php print $language->dir ?>" > <head> <title><?php print $head_title ?></title> <?php print $head ?> <?php print $styles ?> <?php print $scripts ?> <script type="text/javascript"> jQuery.extend(Drupal.settings, <?php print drupal_to_js($views_ajax_settings); ?>); </script> <script type="text/javascript" src="<?php print(base_path() . path_to_theme()); ?>/scripts/scripts.js" ></script> <!--[if lt IE 8 ]><link rel="stylesheet" type="text/css" href="<?php print(base_path() . path_to_theme()); ?>/css/ielt8.css" media="screen" /><![endif]--> </head> <body></body> </html> </html>
Javascript File
/** * Ajax Load Views * See template.php for the data in Drupal.settings.views.ajaxViews **/ $(Drupal.settings.views.ajaxViews).each(function () { var data = {}; // Add view settings to the data. for (var key in this) { data[key] = this[key]; $.ajax({ url: Drupal.settings.views.ajax_path, type: "GET", data: data, success: function (response) { var viewDiv = ".view-dom-id-" + data.view_dom_id; //$('body.front #content .banner').html(response.display); // Call all callbacks. if (response.__callbacks) { $.each(response.__callbacks, function (i, callback) { eval(callback)(viewDiv, response); }); } }, error: function (xhr) {}, dataType: "json", }); } });
The html
<div class="view-dom-id-$view->name preloader"></div>