Client Side Geolocation
By Lance Gliser
Published:
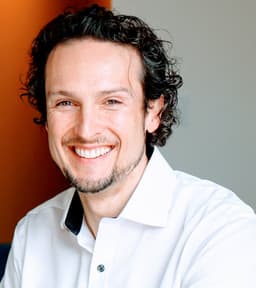
Heads up! This content is more than six months old. Take some time to verify everything still works as expected.
This particular example shows you how to update a version 3 Google map to your current location. But, it should show you the needed pieces to allow you to create your geolocation js effects. Most of the comments you'll need are right in the code for easy copy paste.
// costume module housing object customModule = { // globalInit function should other things be required later init: function(){ // Fire nearby map updates customModule.nearby.init(); } ,nearby: { tracker: null ,minimumAccuracy: 150 // Must report that it is within at least 150 meter accuracy ,map: null // The map object to be updated ,latitude: null // Last known latitude ,longitude: null // Last known longitude ,init: function(){ // Only try to fire the tracker if it's not tracking if( customModule.nearby.tracker == null){ // Set up a watchPosition to constantly monitor the geo location provided by the browser // Full spec for navigator.geolocation can be found here: http://dev.w3.org/geo/api/spec-source.html#geolocation_interface // Note that watchPosition is cheaper on processing power for the end user than polling the current location multiple times // First, check that the Browser is capable // NOTE: !! forces a boolean response if(!!navigator.geolocation){ // Start a tracker customModule.nearby.tracker = navigator.geolocation.watchPosition( customModule.nearby.success // Register a success callback ,customModule.nearby.error // Register an error callback ,{ // Options enableHighAccuracy:true // This is gets you accuracy anywhere from 5-50ish meters ,maximumAge:30000 ,timeout:27000 // How long without gps updates the code should continue to try to fire in milliseconds } ); } else { // Some browsers will not be capable // Others my have capable browsers, with the setting for 'allow websites to ask my location' off // Give a "Please enable geolocation in your browser for more accuracy" jQuery('body').prepend('<div class="warning">Your device is not capable of geolocation. Please see <a href="">this page</a> for ways to turn it on.</div>'); } } } ,success: function(position){ // Ensure we actually have moved. No point spending cpu cycles to update the map if we have not. if( customModule.nearby.latitude != position.coords.latitude || customModule.nearby.longitude != position.coords.longitude ){ // Pan the map to the current location customModule.nearby.map.panTo( new google.maps.LatLng(position.coords.latitude, position.coords.longitude) ); } // Check that the accuracy of our Geo location is sufficient for our needs // If it is, stop running geolocation. // Remove this to cause the device to continually update to your location // One use of this would be to follow you down the street if( position.coords.accuracy <= customModule.nearby.minimumAccuracy ){ navigator.geolocation.clearWatch( customModule.nearby.tracker ); } } ,error: function(error){ // Todo: Decide if you want to announce that errors have occured. The two most common are below switch(error.code){ case 1: // alert('User denied Geolocation'); break; case error.TIMEOUT: // Note that desktops that share their location, will eventually hit this // It's annoying, and maybe should not be stated // Perhaps toggling some 'tracking' on off light on the screen would be better // alert('Timeout!'); break; }; } } } jQuery(document).ready(function(){ customModule.init(); )};