Drupal 7 Services Example - PHP Curl
By Lance Gliser
Published:
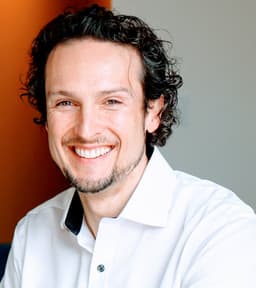
Heads up! This content is more than six months old. Take some time to verify everything still works as expected.
I had a need to consume a custom service I created in Drupal services. There's loads of tutorials on how to do that, feel free to google. But my previous code for using the api I created was for D6, a few things have changed. Looking around I found most examples were javascript, or using Drupal's http library. I wrote a tiny little object just as a simple way to consume using curl for reference. It's not complete, but it lets you log in and out. You can take it from there to build what you need.
/** * Returns an api class for use */ class ApiConsumer { const API_ENDPOINT = '/api'; private static $username = '{name}'; private static $password = '{password}'; private $protocol; private $host_name; private $api_uri; private $connected = FALSE; private $session_cookie; private $token; public function __construct(){ // Define target $this->protocol = PhonyVendorCheck::getTargetProtocol(); $this->host_name = PhonyVendorCheck::getTargetHostName(); $this->api_uri = $this->protocol . $this->host_name . ApiConsumer::API_ENDPOINT . '/'; // Automatically login $this->login(self::$username, self::$password); } function __destruct(){ // Automatically log out $this->logout(); } /** * Determines if the api is connected or not */ public function connected(){ return $this->connected; } /** * Attempts to login a user * @param string $username * @param string $password * @return boolean */ private function login($username, $password){ if( $this->connected ){ return TRUE; } else { $response = $this->request('POST', 'user/login', array( 'username' => $username, 'password' => $password, )); if( empty($response) || empty($response->session_name) || empty($response->sessid) ){ return FALSE; } $this->connected = TRUE; $this->session_cookie = $response->session_name . '=' . $response->sessid; $this->token = $response->token; return TRUE; } } /** * Attempts to logout a user * @param string $username * @param string $password * @return boolean */ private function logout(){ if( !$this->connected ){ return FALSE; } else { $response = $this->request('POST', 'user/logout'); if( empty($response) ){ return FALSE; } $this->connected = FALSE; $this->session_cookie = NULL; $this->token = NULL; return TRUE; } } /** * Makes a request * @param string $method GET * @param string $uri * @param array $data * @return mixed */ private function request($method = 'GET', $uri, $data = array()){ //--------------login to the server------------------------ $service_url = $this->api_uri . $uri . '.json'; // set up the request $curl = curl_init($service_url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE); // have curl_exec return the string if( $method == 'POST' ){ $json_data = json_encode($data); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($curl, CURLOPT_POSTFIELDS, $json_data); $headers = array( 'Content-Type: application/json', 'Content-Length: ' . strlen($json_data), ); if( !empty($this->token) ){ $headers[] = 'X-CSRF-Token:' . $this->token; } curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); } else { $headers = array(); if( !empty($this->token) ){ $headers[] = 'X-CSRF-Token:' . $this->token; } curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); } if( !empty($this->session_cookie) ){ curl_setopt($curl, CURLOPT_COOKIE, $this->session_cookie); } // make the request curl_setopt($curl, CURLOPT_VERBOSE, true); // output to command line try { $response = curl_exec($curl); } catch(Exception $e) { return FALSE; } curl_close($curl); return json_decode($response); } }