Drupal Content Type Module Skeleton
By Lance Gliser
Published:
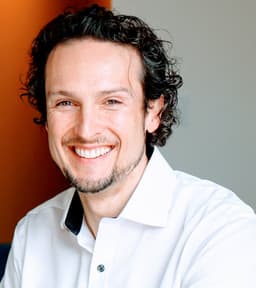
Heads up! This content is more than six months old. Take some time to verify everything still works as expected.
If you're a big fan of CCK, you've probably noticed that there are
limitations eventually. It does fantastic work with data, but not so
much with the nodes themselves. If you need things like access
rights, redirection after XYZ, or custom permissions for business
logic, you will like end up having to create your own content type
through a module. Following is a very simple copy paste you can use
to get started. It's got the most common hooks in there. Just copy
and replace 'node_type' with whatever you wish yours to be. I'll
include a .info
, .install
, and .module
to make things extra
cheesy.
node_type.info
name = Content Type
description = Description
package = Custom
version = VERSION
core = 6.x
node_type.module
node_type.module
/** * - Implementation of hook_node_info */ function node_type_node_info() { return array( 'node_type' => array( 'name' => t('node_type'), 'module' => 'node_type', 'description' => t('Description.'), ) ); } /** * - Implementation of hook_perm */ function node_type_perm() { return array( 'create node_type' ,'edit own node_type' ,'edit any node_type' ,'delete own node_type' ,'delete any node_type' ,'view own node_type' ,'view any node_type' ); } /** * - Implementation of hook_access(). */ function node_type_access($op, $node, $account) { switch ($op) { case 'create': if (user_access('create node_type', $account)) { return TRUE; } break; case 'update': if (user_access('edit any node_type', $account) || ($account->uid == $node->uid && user_access('edit own node_type', $account))) { return TRUE; } break; case 'delete': if (user_access('delete any node_type', $account) || ($account->uid == $node->uid && user_access('delete own node_type', $account))) { return TRUE; } break; case 'view': if (user_access('view any node_type', $account) || ($account->uid == $node->uid && user_access('view own node_type', $account))) { return TRUE; } break; } } /** * - Implementation of hook_form(). */ function node_type_form(&$node, $form_state) { // The site admin can decide if this node type has a title and body, and how // the fields should be labeled. We need to load these settings so we can // build the node form correctly. $type = node_get_types('type', $node); if ($type->has_title) { $form['title'] = array( '#type' => 'textfield', '#title' => check_plain($type->title_label), '#required' => TRUE, '#default_value' => $node->title, '#weight' => -5 ); } if ($type->has_body) { // In Drupal 6, we use node_body_field() to get the body and filter // elements. This replaces the old textarea + filter_form() method of // setting this up. It will also ensure the teaser splitter gets set up // properly. $form['body_field'] = node_body_field($node, $type->body_label, $type->min_word_count); } return $form; } /** * - Implementation of hook_validate() */ function node_type_validate($form, &$form_state) { } /** * - Implementation of hook_view */ function node_type_view($node, $teaser = 0, $page = 0) { $node = node_prepare($node, $teaser); return $node; } /** * - Implementation of hook_insert(). */ function node_type_insert($node) { } /** * - Implementation of hook_update(). */ function node_type_update($node) { } /** * - Implementation of hook_delete(). */ function node_type_delete($node) { }
node_type.install
/** * - Implementation of hook*schema(). */ function node_type_schema() { $schema['node_type'] = array( ); return $schema; } /** * - Implementation of hook_install(). */ function node_type_install() { //drupal_install_schema('node_type'); } /** * - Implementation of hook_uninstall(). */ function node_type_uninstall() { //drupal_uninstall_schema('node_type'); // Advisable to loop over all nodes owned by this module and call node_delete() to clear out system references }