Drupal - Find Nearby Nodes
By Lance Gliser
Published:
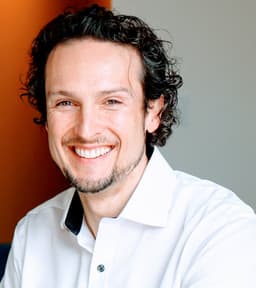
Heads up! This content is more than six months old. Take some time to verify everything still works as expected.
I frequently need to find nodes near a location. The needs vary, but the actual search logic rarely changes. I use this function, along with the installed Location module to find nodes near a coordinate set. Note that this is Drupal 6 code. You'll need to adjust the variable handling for D7.
/** * Finds nodes near a given location based on parameters * * @param array $parameters * $parameters['latitude'] Required - Latitude coordinate to search from * $parameters['longitude'] Required - Latitude coordinate to search from * $parameters['distance'] = 5 Distance in miles to search * $parameters['distance_units'] = 'miles' - Option 'meters' * $parameters['type'] Type of content to search * $parameters['status'] Status of content to search */ function locations_get_nearby_nodes($params){ $params += array( 'distance' => 5, 'distance_units' => 'miles' ); // Distance needs to be converted from miles to meters! if( $params['distance_units'] == 'miles' ){ $params['distance'] = $params['distance'] / 0.000621371192; } // Get location parameters we need $distance_sql = earth_distance_sql($params['longitude'], $params['latitude'], 'location'); $latitude_range = earth_latitude_range($params['longitude'], $params['latitude'], $params['distance']); $longitude_range = earth_longitude_range($params['longitude'], $params['latitude'], $params['distance']); // Setup where logic $where = ''; $query_args = array(); // Location $where .= "location.latitude > %f AND location.latitude < %f AND location.longitude > %f AND location.longitude < %f"; $query_args[] = $latitude_range[0]; $query_args[] = $latitude_range[1]; $query_args[] = $longitude_range[0]; $query_args[] = $longitude_range[1]; // Node type if( array_key_exists( 'type', $params ) ){ $where .= " AND node.type = '%s'"; $query_args[] = $params['type']; } // Node status if( array_key_exists( 'status', $params ) ){ $where .= " AND node.status = %d"; $query_args[] = $params['status']; } $query = "SELECT node.nid ,node.title ,location.lid ,location.latitude ,location.longitude ," . $distance_sql . " AS distance FROM node node JOIN location_instance location_instance ON node.vid = location_instance.vid JOIN location location ON location_instance.lid = location.lid WHERE " . $where . " ORDER BY distance ASC"; $results = db_query($query, $query_args); $locations = array(); while( $result = db_fetch_object($results) ){ if( $params['distance_units'] == 'miles' ){ $result->distance = $result->distance * 0.000621371192; } $result->node = node_load($result->nid); $locations[] = $result; } return $locations; }